Mastering Laravel Queues: An Essential Tool for Optimizing Your Web Applications
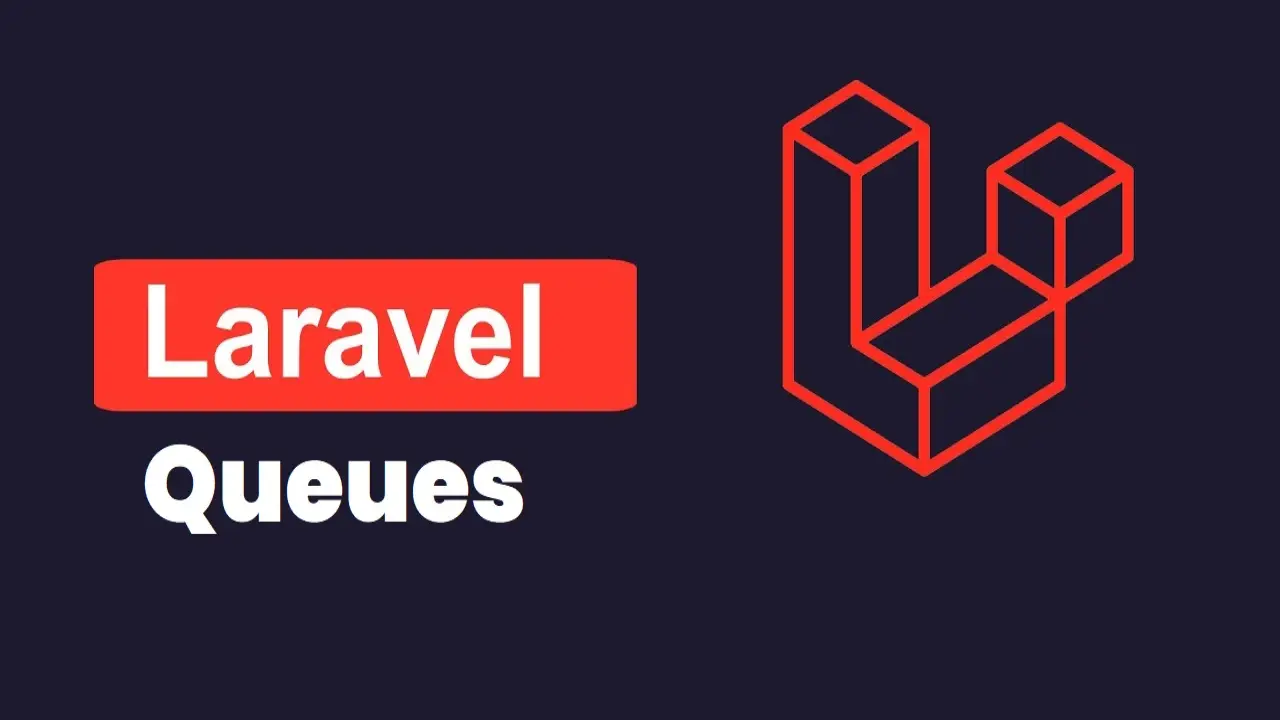
Laravel has become one of the most widely-used PHP frameworks, providing a sleek and efficient approach to web application development. One of the standout features of Laravel is its robust queue system. If you’re working on any application that requires handling tasks asynchronously or processes that could otherwise delay user experience, mastering Laravel queues is crucial.
In this article, we’ll explore the importance of queues in Laravel, how they work, and why they are essential for building high-performing web applications.
What is a Laravel Queue?
A queue in Laravel is a powerful way to defer the processing of a time-consuming task, like sending emails or processing large files, until later. Rather than executing a process immediately and blocking the user’s experience, you can push the task onto a queue for background processing. This helps in improving application performance, as the user doesn’t have to wait for these tasks to finish before they can continue interacting with the application.
Laravel queues offer a unified API across a variety of queue backends, such as:
- Database: The simplest way to get started with queues in Laravel.
- Redis: An in-memory data store that allows for high-performance queue management.
- Amazon SQS: A scalable queuing service provided by AWS.
- Beanstalkd: A simple, fast, and reliable work queue service.
Why Should You Use Laravel Queues?
Here are some compelling reasons to implement queues in your Laravel applications:
- Enhanced User Experience: Without queues, every time-consuming process (like image processing or email sending) would block the user’s interaction with the site. Queues allow these processes to run in the background while users continue using the application, leading to a smoother experience.
- Improved Performance: By offloading time-consuming tasks to the background, you free up resources for the core functionality of your application. This ensures that your app runs faster, especially under high traffic.
- Scalability: With the ability to distribute jobs across multiple servers or processes, Laravel queues offer horizontal scalability. This means that as your application grows, you can scale it to handle more traffic and more background tasks without any performance degradation.
- Error Handling and Retry Logic: Laravel’s queue system includes built-in support for handling failed jobs and retrying them. This ensures that if something goes wrong, the task is not lost and can be retried automatically.
How Laravel Queues Work
Understanding how Laravel handles queues is key to effectively using them. Here’s a simple breakdown of how it works:
- Dispatching Jobs: First, you need to create jobs in your Laravel application. A job is a simple PHP class that encapsulates a task you want to perform. Jobs can be queued using Laravel’s dispatch() function.
- Queue Workers: Once jobs are pushed to the queue, you need workers to process these jobs. Laravel workers listen to a specific queue and process any jobs waiting in it. These workers can be run on the command line using php artisan queue:work.
- Queue Backends: Laravel supports various queue backends, such as Redis, database, Amazon SQS, and others. These backends store the queued jobs and allow for efficient task handling, even when scaling your application.
- Job Handling and Retries: Laravel offers robust error handling. If a job fails, it will be automatically retried based on the configured number of attempts. Additionally, failed jobs can be logged for review.
Setting Up Laravel Queues
To use queues in Laravel, you need to follow a few simple steps:
Configure the Queue Driver: Laravel supports several queue drivers. To get started, configure your .env file with the appropriate queue connection.
QUEUE_CONNECTION=redis
Create Jobs: Create jobs using the artisan command:
php artisan make:job SendWelcomeEmail
Dispatch Jobs: Dispatch the job to the queue:
SendWelcomeEmail::dispatch($user);
Run the Queue Worker: Process jobs with the queue worker:
php artisan queue:work
Monitor and Retry: Use Laravel’s built-in job monitoring to ensure that jobs are processed correctly. If any jobs fail, Laravel will retry them based on your configuration.
Conclusion
Incorporating Laravel queues into your web application workflow is essential for handling tasks that could otherwise slow down your system. By learning how to efficiently manage background tasks, you can create faster, more responsive applications that enhance the user experience and scale with your growing traffic.
Queues are especially powerful for web applications that require heavy lifting in the background, such as email notifications, data imports, and video processing. With Laravel’s elegant queue system, you can ensure that these tasks are executed smoothly without compromising your app’s performance.
By mastering Laravel queues, you’re not just optimizing your app; you’re setting the stage for a scalable, efficient, and high-performance web application that meets the demands of modern users.